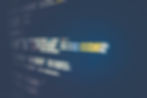
Below is a sample Java JDBC program which takes database connection string as command line arguments and executes the SQL update statement.
Value to be updated in the table is passed as a command line argument.
Replace the SQL update statement in the below code with the desired update statement.
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
public class Run_SQL_Update {
static Connection sqlConn = null;
static Statement sqlStmt = null;
static ResultSet sqlResultset = null;
public static void main(String[] args) throws SQLException {
try {
Class.forName("oracle.jdbc.driver.OracleDriver");
} catch (ClassNotFoundException exception) {
System.out.println("Oracle Driver Class Not found Exception: " + exception.toString());
return;
}
DriverManager.setLoginTimeout(5);
try {
String db_host=args[0];
String db_serviceName=args[1];
String db_username=args[2];
String db_password=args[3];
String jdbc_conn_string="jdbc:oracle:thin:@(DESCRIPTION=(ADDRESS=(PROTOCOL=TCP)(HOST="+db_host+")(PORT=1521))(CONNECT_DATA=(SERVER=DEDICATED)(SERVICE_NAME="+db_serviceName+")))";
sqlConn = DriverManager.getConnection(jdbc_conn_string, db_username, db_password);
}
catch (SQLException e) {
System.out.println("Connection Failed! Check output console");
e.printStackTrace();
System.exit(1);
return;
}
sqlStmt = sqlConn.createStatement();
String var1 = args[4];
String var2 = args[5];
sqlResultset = sqlStmt.executeQuery("update SAMPLE_TABLE SET SAMPLE_COLUMN1='"+var1+"' where SAMPLE_COLUMN2=='"+var2+"'");
sqlResultset.close();
}
}
Copy paste the above code in a file having same name as the class name.
Replace the SQL update statement as per your requirement.
Compile the code:
javac Run_SQL_Update.java
Execute the code with the class name:
java Run_SQL_Update ${DB_HOST} ${DB_SID} ${DB_USER} ${DB_PASSWORD} ${VALUE_TO_UPDATE} ${CONDITION_VALUE}